
- #PYTHON MERGE DICTIONARIES SAME KEYS HOW TO#
- #PYTHON MERGE DICTIONARIES SAME KEYS UPDATE#
- #PYTHON MERGE DICTIONARIES SAME KEYS CODE#
Similarly, the only constraint on the dictionaries’ values is that they can be compared against one another.
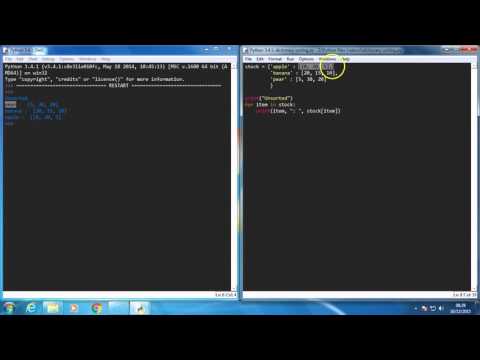
#PYTHON MERGE DICTIONARIES SAME KEYS CODE#
Also note that our code is general: it makes no presumptions about the dictionaries’ keys - they need not be strings nor of any other particular type. This, along with a good docstring, makes our code easy to read, understand, and debug. Note the use of simple and descriptive variables (e.g. we use the variable name key when we iterate over the keys of a dictionary). Parameters - dict1 : Dict dict2 : Dict Returns - Dict The merged dictionary """ merged = dict ( dict1 ) for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged
#PYTHON MERGE DICTIONARIES SAME KEYS HOW TO#
You’ll also learn how to append list values when merging dictionaries. You’ll learn how to combine dictionaries using different operators, as well as how to work with dictionaries that contain the same keys. Thus calling this function will mutate (change) the state of dict1, as demonstrated here:ĭef simple_merge_max_mappings ( dict1, dict2 ): """ Merges two dictionaries based on the largest value in a given mapping. NovemIn this tutorial, you’ll learn how to use Python to merge dictionaries. Recall that dictionaries are mutable objects and that the statement merged = dict1 simply assigns a variable that references dict1 rather than creating a new copy of the dictionary. The problem with our function is that we inadvertently merge dict2 into dict1, rather than merging the two dictionaries into a new dictionary. Please note that we can merge even more than two dicts using this approach.
#PYTHON MERGE DICTIONARIES SAME KEYS UPDATE#
Since we’ve to keep the unit2 values in case of duplicates, so we should update it last. Merged is initialized to have the same mappings as dict1, this is a correct algorithm for merging our two dictionaries based on max-value. The most obvious way to combine the given dictionaries is to call the update () method for each unit. Moreover, the values of the common keys will be merged together in one list. We then set a key-value from dict2 mapping in merged if that key doesn’t exist in merged or if the value is larger than the one stored in existing mapping. The returned dictionary should contain all the keys of the passed dictionaries. But what if both the dictionaries contain a similar key In such situations. Thus for key in dict2 loops over every key in dict2. Dictionary merges are typically performed from right to left, as dict a <- dict b. Recall that iterating over a dictionary will produce each of its keys one-by-one. The first one is the dictionary and the second one is a closure that takes the current and new value for any duplicate key and. Let’s first see what this function does right. To handle the duplicate keys, the idea remains the same group elements based on. Then, we create a new variable (called new) and assign the dictionary with d3,d4 parameters using the exponential operator (basically we update with the methods from the d3 and d4 dictionary).Def buggy_merge_max_mappings ( dict1, dict2 ): # create the output dictionary, which contains all # the mappings from `dict1` merged = dict1 # populate `merged` with the mappings in dict2 if: # - the key doesn't exist in `merged` # - the value in dict2 is larger for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged The above code throws an ArgumentException if it reencounters the same key.Then, using the update method, we update d2 with values of d1, basically merging the two dictionary items as updated elements.Next, the update method ( d1) with the values of d2 merge and the two dictionaries’ items are updated.Next, we initialize a variable ( d4) and give it the dictionary ( d2).Then, we create dictionary d1 with key values 4,5,6 and other fruits as values.Next, we initialize a variable ( d3) and give it the same value as the dictionary ( d1).Note: If there are two keys with the same name, the merged dictionary. First, we create a dictionary ( d1) with the values set as fruit names. In Python 3.9 and later versions, the operator can be used to merge dictionaries.
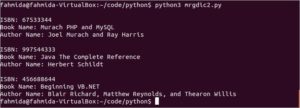
Values can be of any data type and duplicated, whereas keys can’t be repeated and must be immutable. A dictionary is a collection of key-value pairs or items that can be referred to using a key name.ĭuplicate items are unordered items do not have a specific order, changeable, and do not allow duplicates.
